This SQLite Java section guides you step-by-step through how to interact with SQLite using Java JDBC API.
Several interfaces are available for interacting with SQLite in Java. Some provide a wrapper around the native C API, while others implement the standard Java Database Connectivity (JDBC) API.
In this section, we will introduce you to the modern SQLiteJDBC package, a JDBC driver for SQLite. The SQLiteJDBC
package includes Java classes and native SQLite libraries for Windows, macOS, and Linux.
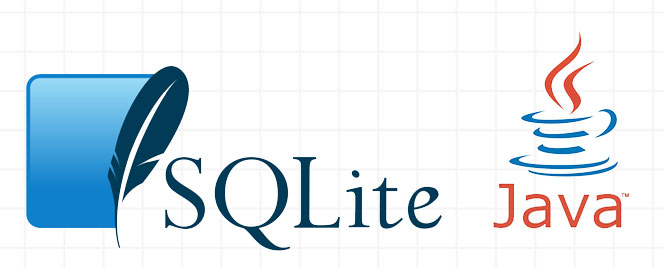
Section 1. Connecting to SQLite
- Connecting to an SQLite database – Show you how to download the
SQLiteJDBC
driver and connect to a SQLite database using JDBC. - Creating a new SQLite database – Show you how to create a new SQLite database from a Java program using
SQLiteJDBC
driver.
Section 2. Creating Tables
- Creating a new table using JDBC – Show you how to create a new table in an SQLite database from a Java program.
Section 3. CRUD
- Inserting data into a table from a Java program – Walk you through the steps for inserting data into a table from a Java program
- Querying data from a table with or without parameters – Query data using a SELECT statement. You will learn how to issue a simple
SELECT
statement to query all rows from a table and use a query with parameters to select data based on the user’s input. - Updating existing data using the PreparedStatement object – Guide you on updating data in a table in an SQLite database from a Java program.
- Deleting data from a table – Provide the steps for deleting one or more rows from a table in a Java program.
Section 4. Managing Transactions
- Managing transactions – Manage SQLite transactions using Java JDBC API such as
setAutoCommit
,commit
, androllback
.
Section 5. Handling BLOB data
- Writing and Reading SQLite BLOB – Show you how to update the SQLite BLOB data into a table and query BLOB data for displaying.